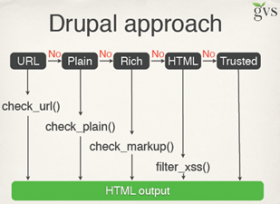
A3 XSS (Cross Site Scripting)
XSS flaws occur whenever an application takes untrusted data and sends it to a web browser without proper validation or escaping. XSS allows attackers to execute scripts in the victim’s browser which can hijack user sessions, deface web sites, or redirect the user to malicious sites.
XSS: XSS is injecting data to the HTML output. This is a very important one: there is a 64% likelihood a website has a XSS issue. You should always escape the variables you send to the output if they come from a non trusted source, like the parameters of a URL. For example, this code is unsecure:
index.php?id=12
print $_GET['id'];
It's also very common see codes like this, specially in custom themes:
$output .= $node->title;
The problem in the code above is that Drupal doesn't usually filter the user input when a node is saved. So the user could save malicious code in the title and then it will be printed without any kind of filtering.
One more obvious mistake is giving full HTML permissions to not trusted users, or allow to use unsafe tags as <script>. If we grant Full HTML permissions to users, they could add this JS code to a page, which would change the admin password if he views the content:
jQuery.get('/user/1/edit',
function (data, status)
{ if (status == 'success') {
var p = /id="edit-user-edit-form-token" value="([a-z0-9]*)"/;
var matches = data.match(p);
var token = matches[1];
var payload = {
"form_id": 'user_edit',
"form_token": token,
"pass[pass1]": 'hacked',
"pass[pass2]": 'hacked'
};
jQuery.post('/user/1/edit', payload);
}
}
);
This technique, with code changes, works up to Drupal 6.
Drupal provides a set of functions you should use:
· PHP: use placeholder in functions like t() or format_plural(): %name, @url, !insecure:
t(' %name has a blog at <a href=" @url " _fcksavedurl=" @url " _fcksavedurl=" @url " _fcksavedurl=" @url "> @url </a>', array('@url' => valid_url($user->profile_blog), '%name' => $user->name));
· JavaScript: Use Drupal.t() , Drupal.formatPlural()
A4 Insecure Direct Object Reference
A direct object reference occurs when a developer exposes a reference to an internal implementation object, such as a file, directory, or database key. Without an access control check or other protection, attackers can manipulate these references to access unauthorized data.
Check whether the user has permission to access objects:
index.php?id= 12 db_query("SELECT * FROM {node} WHERE nid = :id", array(':id' => $_GET['id'] ));
When using Views, make sure the user has access to nodes. Remember to add "published = Yes" to the view filters. Drupal approach:
· Menu system handles permission checking
· user_access('administer nodes', $account)
· node_access('edit', $node, $account);
· $select->addtag('node_access');
· Form API checks for data validity
Comments
Drupal vs OWASP TopTen
Drupal vs OWASP TopTen (continued)